Install navigation
Our app is going to have an onboarding step: when launching for app the first time the user will see a full-screen blocking modal until they enter some info. Note that you could use the same kind of UI pattern if your app had an authentication step.
We will be using Expo Router for navigation. It's a file-system based navigation library, built on top of React Navigation.
Let's open the getting started docs:
1. Install the libraries:
npx expo install expo-router react-native-safe-area-context react-native-screens expo-linking expo-constants expo-status-bar
2. Set up the entry point
In your package.json change the entry point to "main": "expo-router/entry"
.
3. Modify project config
Add the scheme
to your app.json
:
{
"expo": {
"scheme": "plantly"
}
}
A scheme is similar to a url. The reason this is required is because expo router comes with deep linking built in.
4. Set up web (optional)
Install the react-native-web
and react-dom
for web support.
npx expo install react-native-web react-dom
5. Set bundler to metro (only if setting up)
In your app.json
, set the web bundler to be metro:
{
"web": {
"favicon": "./assets/favicon.png",
+ "bundler": "metro"
}
}
6. Move existing code
Create an /app
folder.
Rename App.tsx
-> index.tsx
and move it to /app/index.tsx
7. Restart the bundler
Restart the bundler with npx expo start --reset-cache
.
Now at this point, if you reload the app it should look exactly the same as before.
Let's finish it off by adding our screen to a stack.
8. Create a _layout
file
Create a _layout.tsx
file in the same directory as the index
file and add a stack layout.
This will add a header using the filename - index
- by default, so we'll override with a custom file name.
New file: app/_layout.tsx
import { Stack } from "expo-router";
export default function Layout() {
return (
<Stack>
<Stack.Screen name="index" options={{ title: "Home" }} />
</Stack>
);
}
9. Check everything works
On iOS and Andorid, check that the app loads with a "Home" header.
Press w
with the terminal open to launch the app in the web.
Checkpoint
Android | iOS |
---|---|
![]() | ![]() |
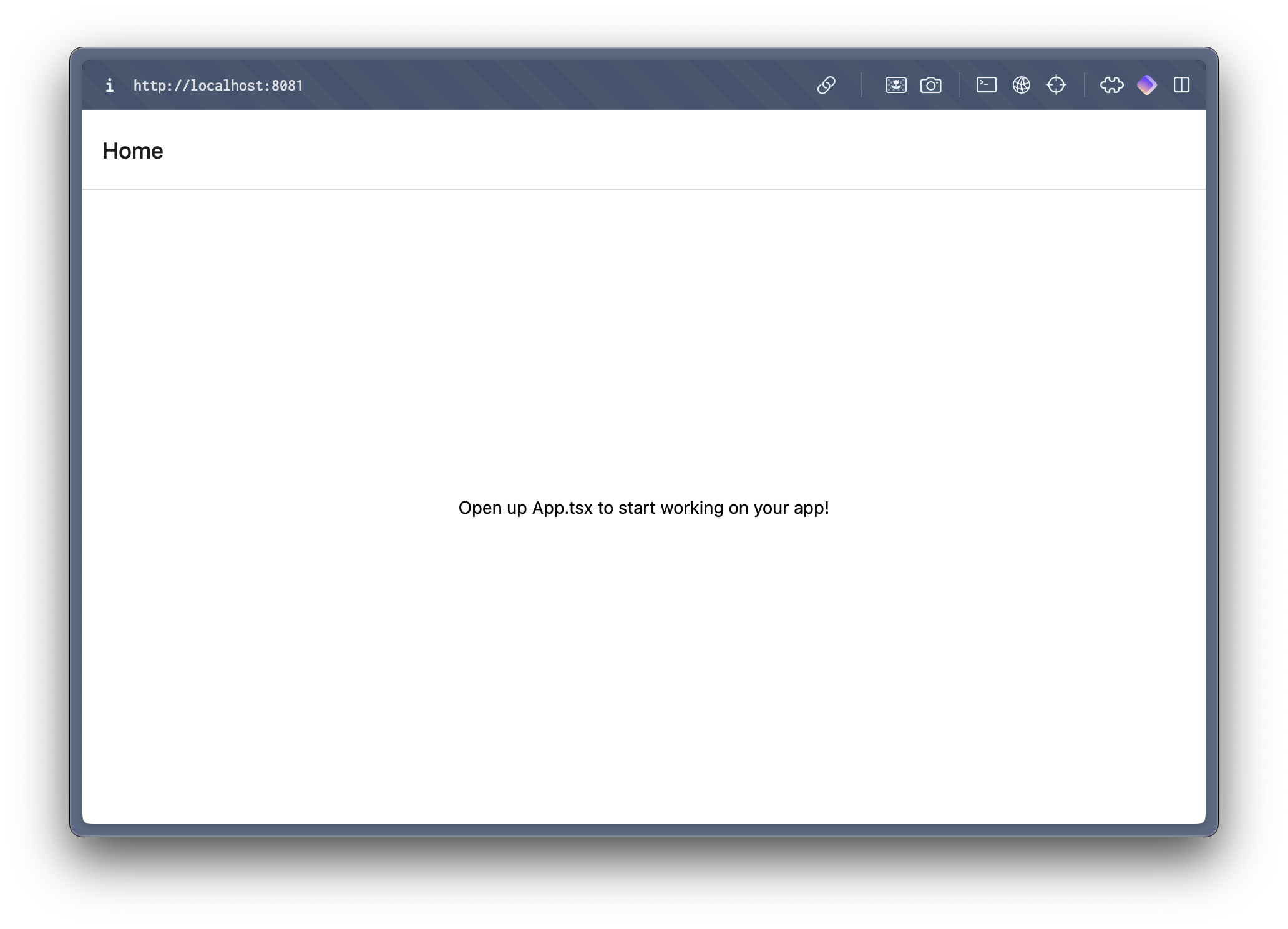
If you're getting an error like PluginError: Failed to resolve plugin for module "expo-router"
, make sure you've installed the libraries from step 1, closed your existing JavaScript bundler process and restarted it with npx expo start --reset-cache