Local image
To finish off the onboarding screen, let's add an image and some text.
We'll use the built-in Image component for this. For advanced usage, you may want to use Expo Image or Fast Image.
For local images in React Native, the image uri is passed in via require
. This ensures the image files are bundled after.
Add the image
Download image from GitHub.
Let's create a new PlantlyImage
component and use the useWindowDimensions
hook to have it render as a consistent ratio of the screen width.
New file: components/PlantlyImage.tsx
import { Image, useWindowDimensions } from "react-native";
export function PlantlyImage() {
const { width } = useWindowDimensions();
const imageSize = Math.min(width / 1.5, 400);
return (
<Image
source={require("@/assets/plantly.png")}
style={{ width: imageSize, height: imageSize }}
/>
);
}
And use the new image component in the onboarding screen:
Update: app/onboarding.tsx
@@ -1,10 +1,11 @@
-import { StyleSheet } from "react-native";
+import { Image, StyleSheet } from "react-native";
import { theme } from "@/theme";
import { useUserStore } from "@/store/userStore";
import { useRouter } from "expo-router";
import { PlantlyButton } from "@/components/PlantlyButton";
import { LinearGradient } from "expo-linear-gradient";
import { StatusBar } from "expo-status-bar";
+import { PlantlyImage } from "@/components/PlantlyImage";
export default function OnboardingScreen() {
const router = useRouter();
@@ -23,6 +24,7 @@ export default function OnboardingScreen() {
colors={[theme.colorGreen, theme.colorAppleGreen, theme.colorLimeGreen]}
>
<StatusBar style="light" />
+ <PlantlyImage />
<PlantlyButton title="Let me in!" onPress={handlePress} />
</LinearGradient>
);
Greeting text
As a final touch, let's add some text in way of welcoming the user into the app.
Update: app/onboarding.tsx
@@ -1,10 +1,11 @@
-import { StyleSheet } from "react-native";
+import { StyleSheet, Text, View } from "react-native";
import { theme } from "@/theme";
import { useUserStore } from "@/store/userStore";
import { useRouter } from "expo-router";
import { PlantlyButton } from "@/components/PlantlyButton";
import { LinearGradient } from "expo-linear-gradient";
import { StatusBar } from "expo-status-bar";
+import { PlantlyImage } from "@/components/PlantlyImage";
export default function OnboardingScreen() {
const router = useRouter();
@@ -23,6 +24,13 @@ export default function OnboardingScreen() {
style={styles.container}
>
<StatusBar style="light" />
+ <View>
+ <Text style={styles.heading}>Plantly</Text>
+ <Text style={styles.tagline}>
+ Keep your plants healthy and hydrated
+ </Text>
+ </View>
+ <PlantlyImage />
<PlantlyButton title="Let me in!" onPress={handlePress} />
</LinearGradient>
);
@@ -31,8 +39,20 @@ export default function OnboardingScreen() {
const styles = StyleSheet.create({
container: {
flex: 1,
- justifyContent: "center",
+ justifyContent: "space-evenly",
alignItems: "center",
backgroundColor: theme.colorWhite,
},
+ heading: {
+ fontSize: 42,
+ color: theme.colorWhite,
+ fontWeight: "bold",
+ marginBottom: 12,
+ textAlign: "center",
+ },
+ tagline: {
+ fontSize: 24,
+ color: theme.colorWhite,
+ textAlign: "center",
+ },
});
Checkpoint
Android | iOS |
---|---|
![]() | ![]() |
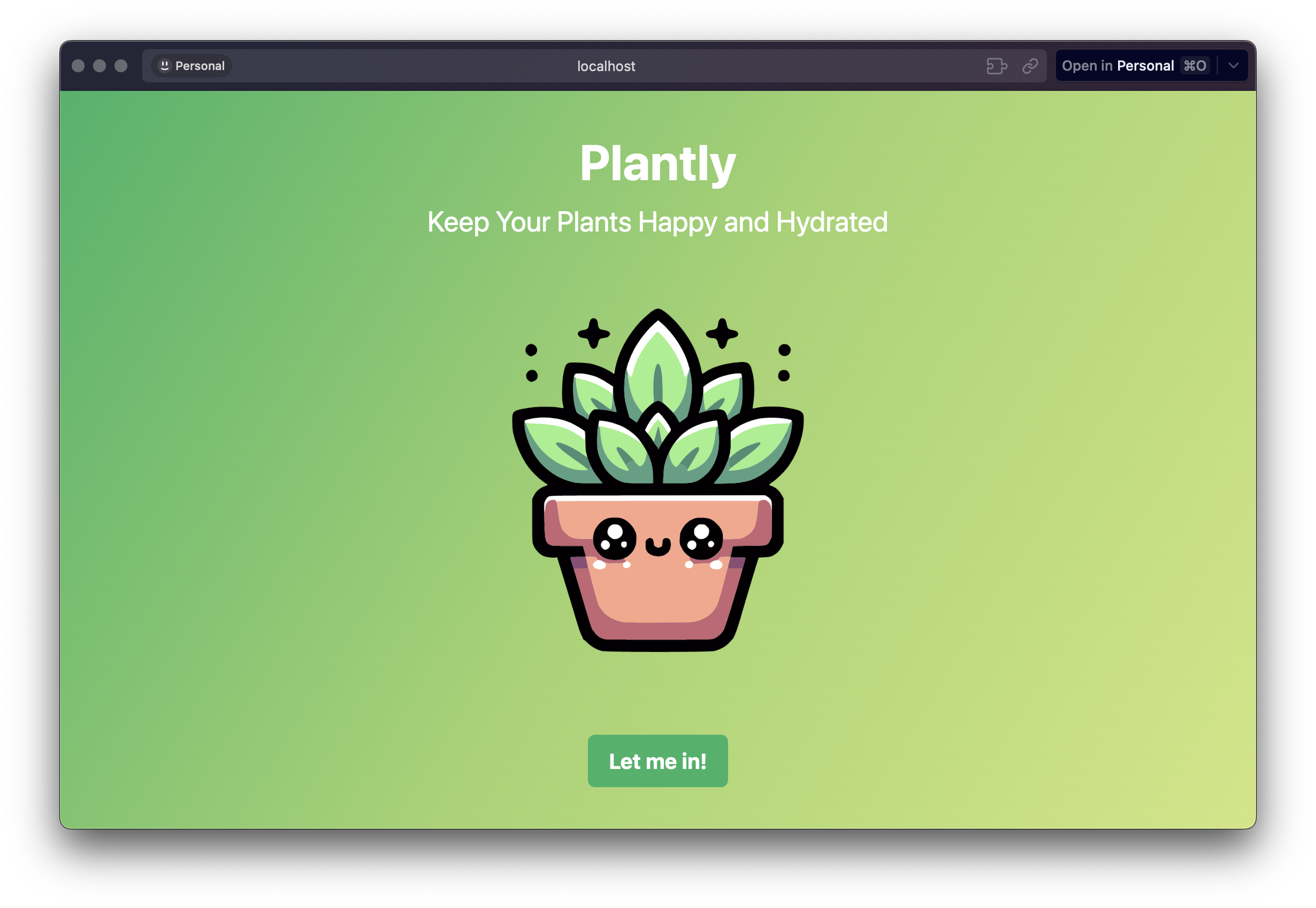
Checkpoint