View and Text
Hopefully, at this point you'll already be quite familiar with View
and Text
:
View
is a container to use for styling and positioning the elements within. Similar todiv
in web development.Text
is used to display text.
Let's use these components to display a emoji in a horizontal list.
const moodOptions = [ { emoji: '๐งโ๐ป', description: 'studious' }, { emoji: '๐ค', description: 'pensive' }, { emoji: '๐', description: 'happy' }, { emoji: '๐ฅณ', description: 'celebratory' }, { emoji: '๐ค', description: 'frustrated' },];
To do this, let's create a components
directory in src
, next to screens
. This will be the place for all our components.
Let's create a new file called MoodPicker.tsx
.
src/components/MoodPicker.tsx
import React from 'react';import { View, Text } from 'react-native';
export const MoodPicker: React.FC = () => { return ( <View> <Text>MoodPicker</Text> </View> );};
And let's update Home.screen.tsx
to display it:
src/screens/Home.screen.tsx
import * as React from 'react';-import { StyleSheet, View, Text } from 'react-native';+import { StyleSheet, View } from 'react-native';+import { MoodPicker } from '../components/MoodPicker';
export const Home: React.FC = () => { return ( <View style={styles.container}>- <Text>Home</Text>+ <MoodPicker /> </View> );};
const styles = StyleSheet.create({ container: { flex: 1,+ justifyContent: 'center', },});
Now let's update the MoodPicker
to display our list of emoji:
src/components/MoodPicker.tsx
import React from 'react';import { View, Text, StyleSheet } from 'react-native';
const moodOptions = [ { emoji: '๐งโ๐ป', description: 'studious' }, { emoji: '๐ค', description: 'pensive' }, { emoji: '๐', description: 'happy' }, { emoji: '๐ฅณ', description: 'celebratory' }, { emoji: '๐ค', description: 'frustrated' },];
export const MoodPicker: React.FC = () => { return ( <View style={styles.moodList}> {moodOptions.map((option) => ( <Text key={option.emoji} style={styles.moodText}> {option.emoji} </Text> ))} </View> );};
const styles = StyleSheet.create({ moodText: { fontSize: 24, }, moodList: { flexDirection: 'row', justifyContent: 'space-between', padding: 20, },});
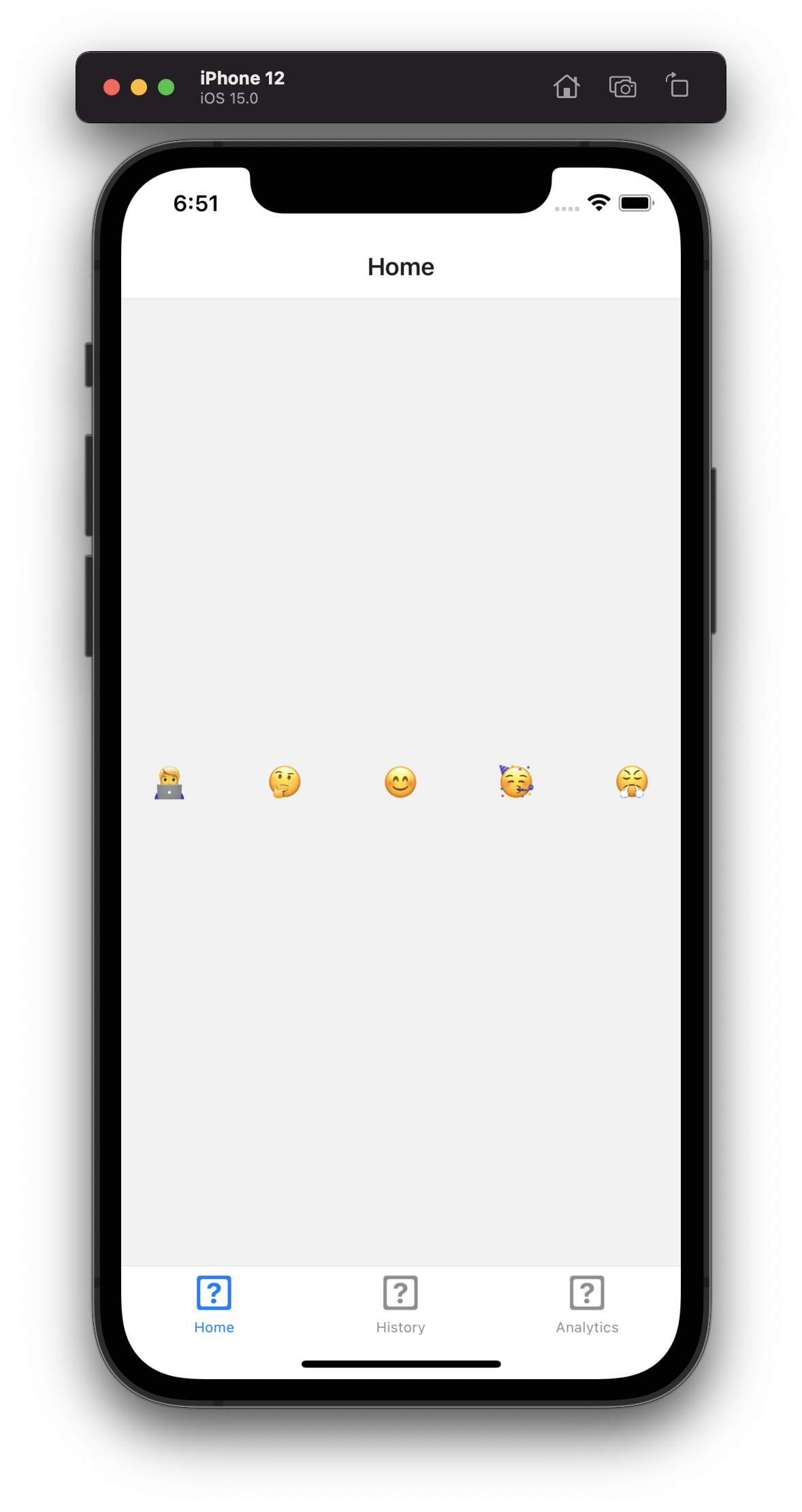
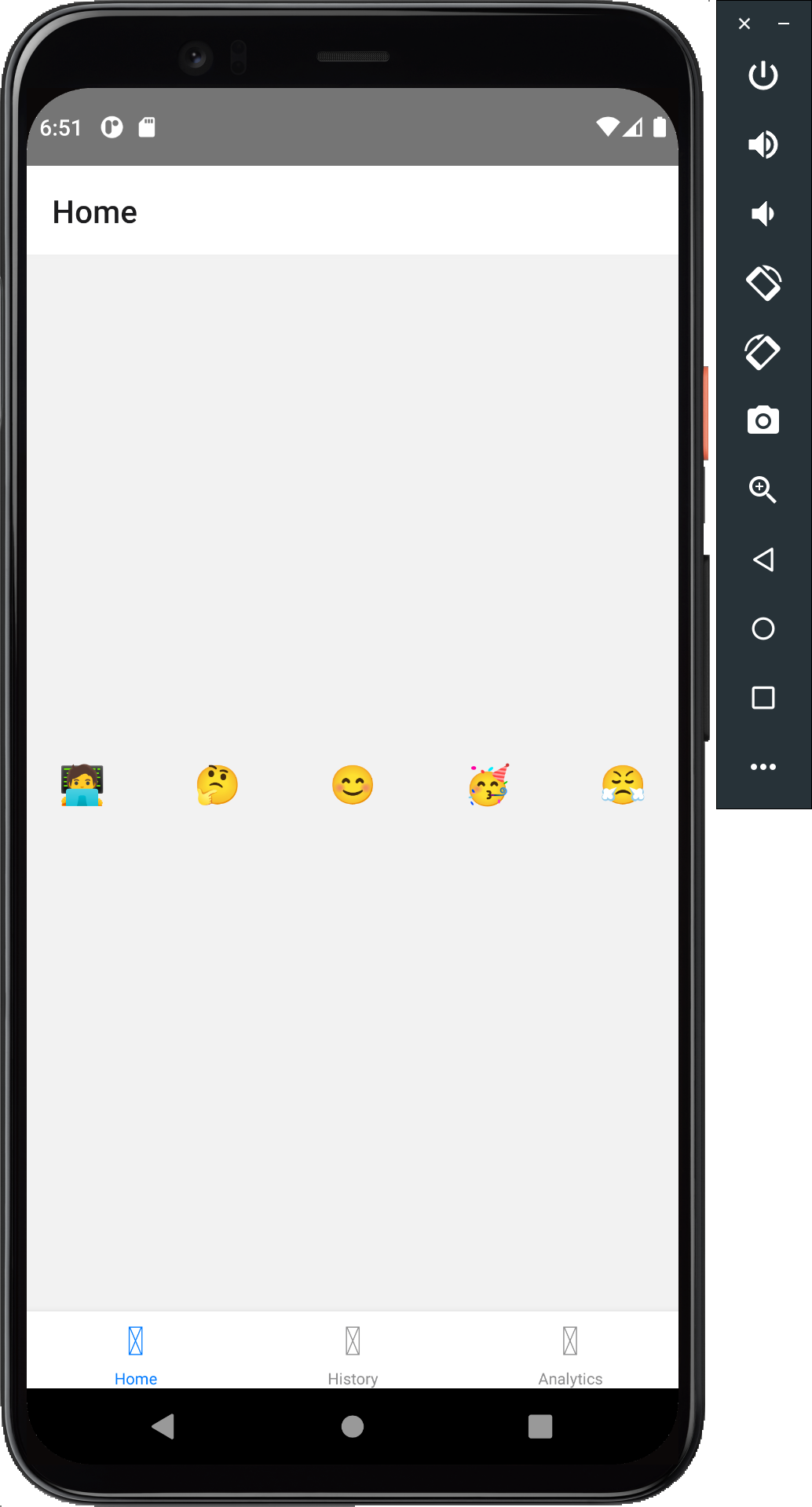