JavaScript Libraries
One of the benefits of React Native is that since we're using JavaScript, we're able to leverage the vast amount of JavaScript community libraries for our project. Not all JavaScript libraries will work on React Native, for instance libraries that rely on the browser environment and interact with the browser window or CSS would have no place here. But there are still a huge amount of various utility libraries that are compatible.
Let's use a JavaScript library to format the date in our history.
I like to use date-fns which includes a lot of handy date-related utility functions, including a format function.
To install the library, open your terminal and run:
yarn add date-fns# ornpm install date-fns
Unlike the navigation library we installed earlier, this library is JavaScript only, meaning there's no need for us to install pods or even rebuild the app. It will be available immediately.
tip
When installing libraries, you have to run pod install
and rebuild your project only if the library includes native code. Plain JavaScript libraries don't include native code and so don't require a rebuild.
Let's make our dates follow the following format: 29 Sep, 2021 at 9:45am
.
You can use the format docs as a reference.
import format from 'date-fns/format';
//
format(new Date(item.timestamp), "dd MMM, yyyy 'at' h:mmaaa");
//
We also have a new colour to use for styling, let's add it to our theme file:
colorLavender: '#87677B',
Let's pull the MoodItemRow
into a separate component and style it accordingly:
import React from 'react';import { View, Text, StyleSheet } from 'react-native';import format from 'date-fns/format';import { MoodOptionWithTimestamp } from '../types';import { theme } from '../theme';
type MoodItemRowProps = { item: MoodOptionWithTimestamp,};
export const MoodItemRow: React.FC<MoodItemRowProps> = ({ item }) => { return ( <View style={styles.moodItem}> <View style={styles.iconAndDescription}> <Text style={styles.moodValue}>{item.mood.emoji}</Text> <Text style={styles.moodDescription}>{item.mood.description}</Text> </View> <Text style={styles.moodDate}> {format(new Date(item.timestamp), "dd MMM, yyyy 'at' h:mmaaa")} </Text> </View> );};
const styles = StyleSheet.create({ moodValue: { textAlign: 'center', fontSize: 40, marginRight: 10, }, moodDate: { textAlign: 'center', color: theme.colorLavender, }, moodItem: { backgroundColor: 'white', marginBottom: 10, padding: 10, flexDirection: 'row', justifyContent: 'space-between', alignItems: 'center', }, moodDescription: { fontSize: 18, color: theme.colorPurple, fontWeight: 'bold', }, iconAndDescription: { flexDirection: 'row', alignItems: 'center', },});
We'll also need to update the Home.screen
to use the new component:
+import { MoodItemRow } from '../components/MoodItemRow';
- <Text key={item.timestamp}>- {item.mood.emoji} {new Date(item.timestamp).toString()}- </Text>+ <MoodItemRow item={item} key={item.timestamp} />
Checkpoint ๐
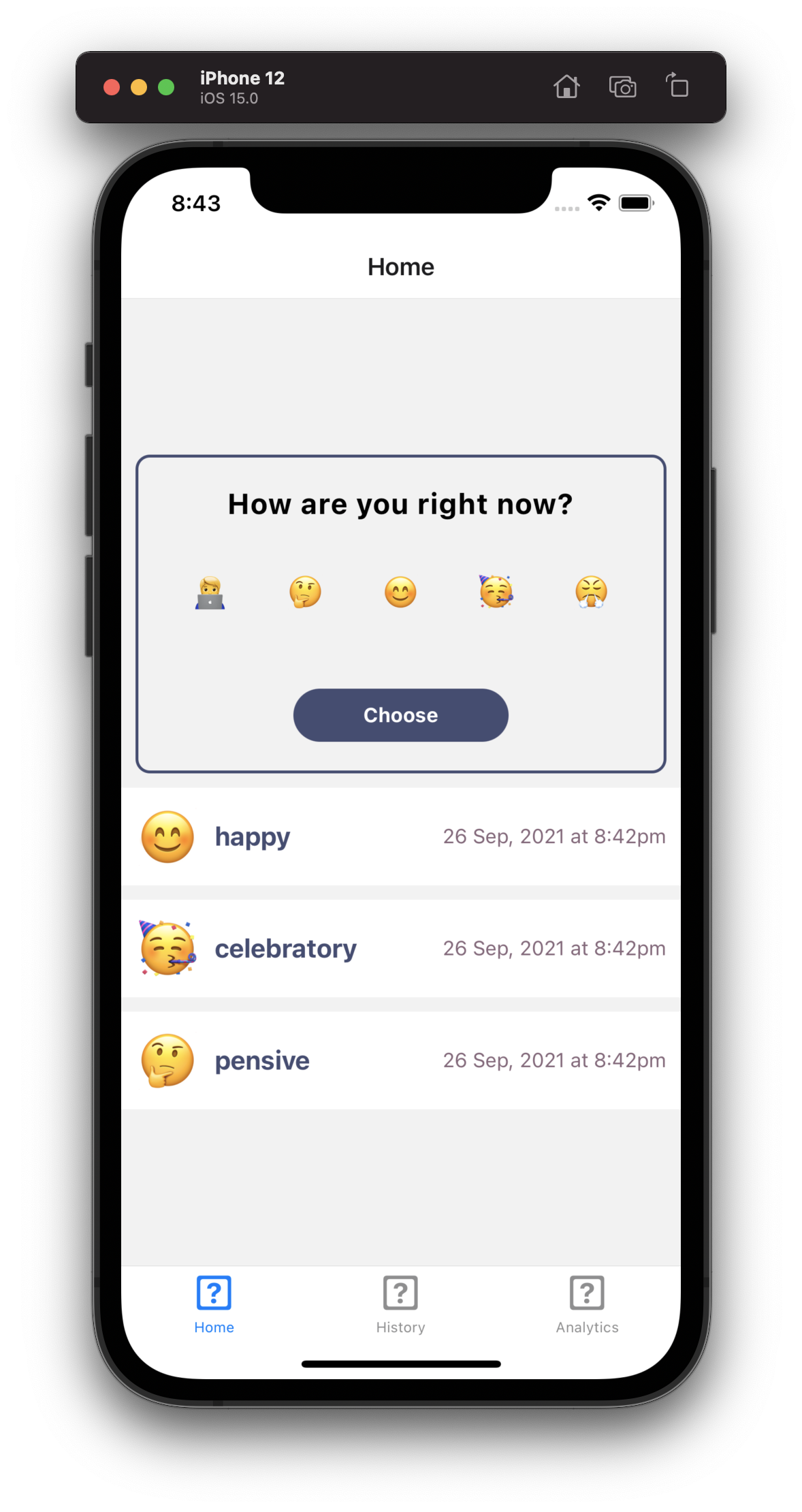
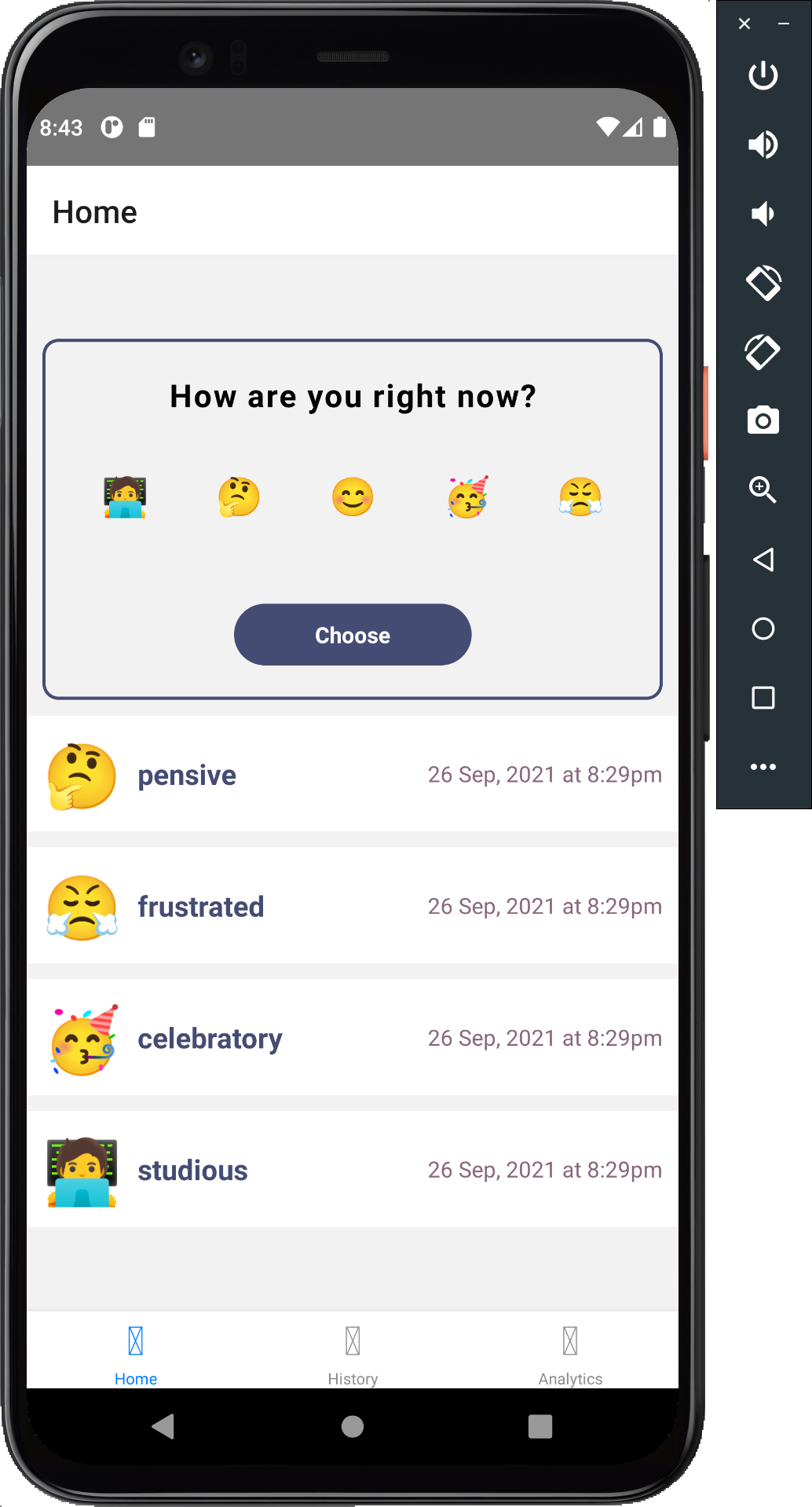