Network Images
By network images, we mean images that are loaded from a url. Usually, most of the images you'll be using in your app are loaded in this way rather than packaged within the app.
In order to render images from a url, let's display this peaceful smokey background for our home page:
Photo from Unsplash
Open the Home.screen.tsx
fine again, and add a constant at the top of the file for the image url:
const imageUrl = 'https://images.unsplash.com/photo-1474540412665-1cdae210ae6b?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=1766&q=80';
Now let's add an image component
<Image source={{ uri: imageUrl }} />
And... nothing renders! That's because for network images, we also need to explicitly tell it how large to make the image. In out case, let's make it 200 pt high:
<Image source={{ uri: imageUrl }} style={{ height: 200 }} />
Voila! Finally, let's move the image to the back of the page so that the whole page is wrapped in the image. Unfortunately the Image
component doesn't allow child components. So in order to do that we must replace it with the ImageBackground
component.
import React from 'react';-import { StyleSheet, View } from 'react-native';+import { StyleSheet, ImageBackground } from 'react-native'; import { useAppContext } from '../App.provider'; import { MoodPicker } from '../components/MoodPicker';+const imageUrl =+ 'https://images.unsplash.com/photo-1474540412665-1cdae210ae6b?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=1766&q=80';+ export const Home: React.FC = () => { const appContext = useAppContext(); return (- <View style={styles.container}>+ <ImageBackground source={{ uri: imageUrl }} style={styles.container}> <MoodPicker onSelect={appContext.handleSelectMood} />- </View>+ </ImageBackground> ); };
Finally, let's tweak the the styles of out MoodPicker
to make the mood picker stand out more on the image:
- add
backgroundColor: 'rgba(0,0,0,0.2)'
to thecontainer
style, and - add
color: theme.colorWhite,
to theheading
style
tip
For production apps, it is recommended to use react-native-fast-image for images that are loaded via url - it's a drop-in replacement for the React Native image component, and it adds a lot of performance optimizations the Image
and ImageBackground
component are missing.
#
Checkpoint ๐Show a background image with ImageBackground da149e5545e90a99ea33439feddcdf3d56b85224
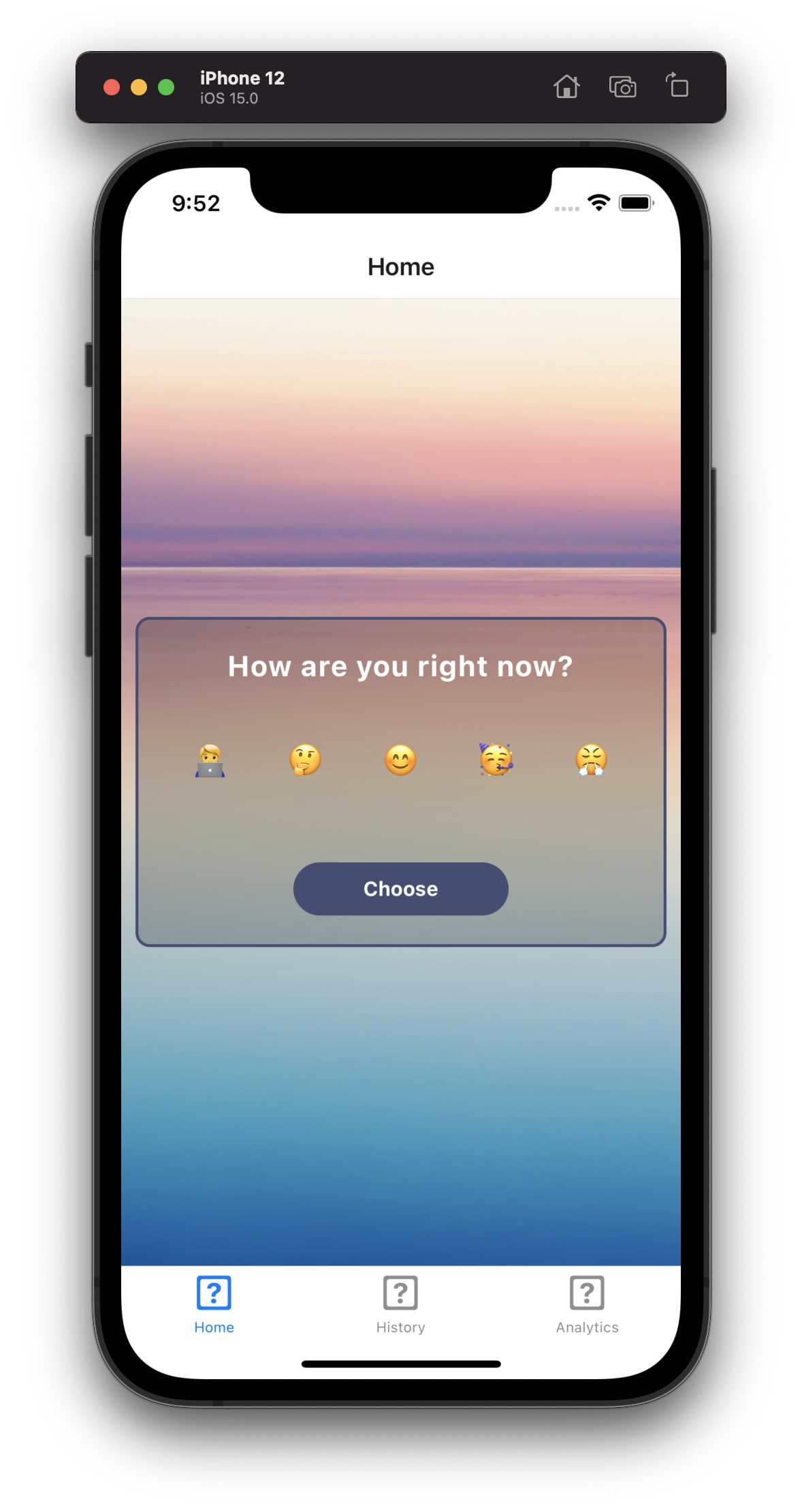
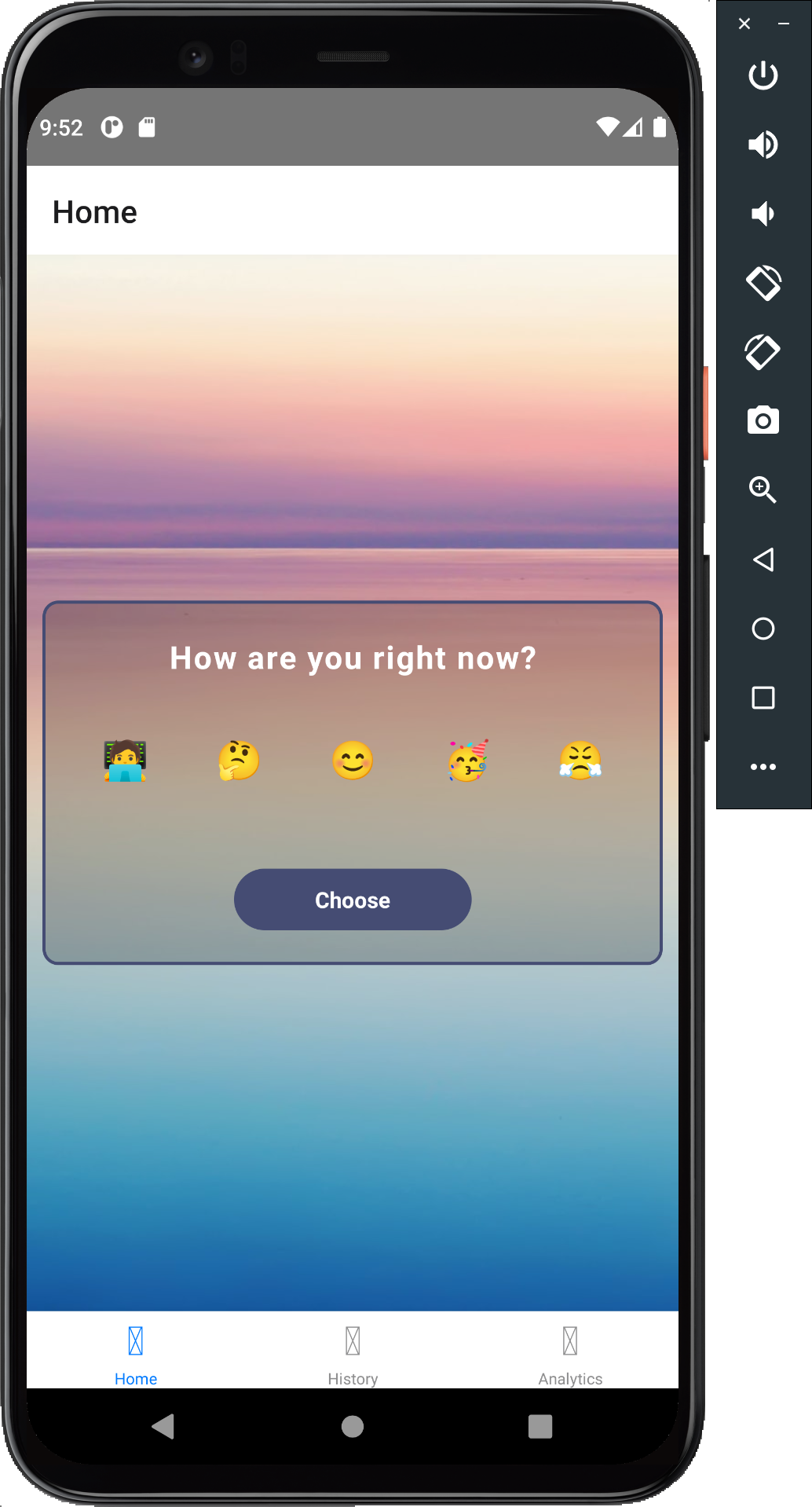