Navigation Exercise 📝
You can pass down parameters to the screen you navigate to as a second argument:
const COLORS = [...];
navigation.navigate('ColorPalette', { paletteName: 'Solarized', colors: COLORS });
This will make the paletteName and colors available to the ColorPalette via the route
prop:
// ColorPalette.js
console.log(route.params.paletteName);
// logs out: Solarized
For this exercise:
- update the app so that the colors and name are being passed into the ColorPalette component, making it reusable. Docs
- make sure the page title will be the name of the color palette instead of the name of the page. Docs
- add two more color schemes: Rainbow and Frontend Masters (hint: you create a
COLOR_PALETTES
array and use aFlatList
to render them) - update the Home page to display the first 5 colors of the color scheme as preview (stretch goal)
const RAINBOW = [
{ colorName: 'Red', hexCode: '#FF0000' },
{ colorName: 'Orange', hexCode: '#FF7F00' },
{ colorName: 'Yellow', hexCode: '#FFFF00' },
{ colorName: 'Green', hexCode: '#00FF00' },
{ colorName: 'Violet', hexCode: '#8B00FF' },
];
const FRONTEND_MASTERS = [
{ colorName: 'Red', hexCode: '#c02d28' },
{ colorName: 'Black', hexCode: '#3e3e3e' },
{ colorName: 'Grey', hexCode: '#8a8a8a' },
{ colorName: 'White', hexCode: '#ffffff' },
{ colorName: 'Orange', hexCode: '#e66225' },
];
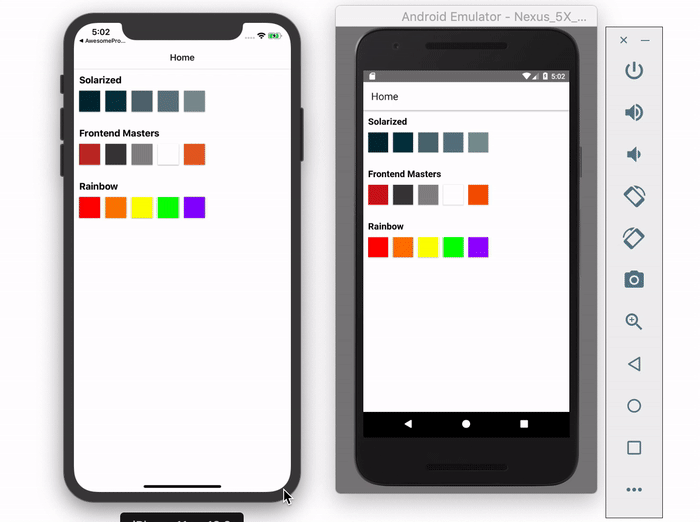
This is a guide for what the completed exercise should looks like. Notice that the navigation is different between iOS and Android? This is because both platforms leverage the native conventions for navigation. It is what the user is expecting and provides the best experience.